CS145 – PROGRAMMING ASSIGNMENT #1 - OVERVIEW This is a review exercise, so the primary goal of the exercise is to get your mind working and in the correct space. In this activity you will create an imaginary cube of locations that goes from -5< x< 5, -5 < y< 5, and –5 < z< 5 with the coordinate (0,0,0) being "home". On this grid you will keep track of various bird objects as they move around the grid. Make sure that all birds stay within that box at all times including when they are created.
CS145 – PROGRAMMING ASSIGNMENT #1 - OVERVIEW This is a review exercise, so the primary goal of the exercise is to get your mind working and in the correct space. In this activity you will create an imaginary cube of locations that goes from -5< x< 5, -5 < y< 5, and –5 < z< 5 with the coordinate (0,0,0) being "home". On this grid you will keep track of various bird objects as they move around the grid. Make sure that all birds stay within that box at all times including when they are created.
Chapter7: Using Methods
Section: Chapter Questions
Problem 20RQ
Related questions
Question

Transcribed Image Text:CS145 – PROGRAMMING ASSIGNMENT #1
OVERVIEW
This is a review exercise, so the primary goal of the exercise is to get your mind working and in
the correct space.
In this activity you will create an imaginary cube of locations that goes from -5 < x < 5, -5 <
y< 5, and -5 <z< 5 with the coordinate (0,0,0) being "home". On this grid you will keep
track of various bird objects as they move around the grid. Make sure that all birds stay within
that box at all times including when they are created.
Using Java, create the following classes and primary program that uses the classes that you
developed.
INSTRUCTIONS
Create the following classes.
BIRD CLASS
Create a Bird class. Each bird has a name, an x,y, and z integer coordinate. The Bird class
should have at minimum the following methods below but you may want to add more if
necessary:
A default constructor that starts the bird at 1,1,1 with a name of "Unknown Bird".
A constructor that allows the user to start with a given name.
In this case, the constructor should give the bird a random x,y,z that are inside
the box.
A parameter constructor that allows the programmer to input all 4 pieces of
information. (x,y,z,name)
Check the parameters for valid input based on the constraints. If any of the
input coordinates is invalid, that particular coordinate should be set to -1.
getX()
getY()
getZ()
getName( )
tostring()
This should print out the name and coordinates of the animal.
touching (Bird other)
This method should determine if the bird is on the same spot as a second bird
(x). It should return a boolean value of true if they are touching and false if they
are not on the same location.
![move()
When this method is run, the animal will move in a random direction.
The animal can move in one of six directions.
The animal could move left, or right, or up, or down, or in/out.
If the animal moves in one of these directions, it should move
random x number of units, where x is between 1 and 2 (inclusive).
Each direction should have an equal chance of happening, so make sure
everything is balanced.
Note that there are no parameters. The animal should self move.
Check for the range constraints.
• After the bird has moved, double check that it is still inside the box.
• If it isn't, undo the move and pick a different direction to move.
Check both x, y, and z coordinates at all times including
construction.
After the bird has moved, double check that it is still inside the box.
MAIN CLASS
Inside your main class do the following
Create an array that can hold 7 birds.
Fill the array with 4 birds with names and locations of your choice.
o Fill the array with 2 named birds but with random locations.
Finish filling the array with one default bird.
Create a touching counter that starts at zero and a round counter.
Print out the starting locations and names of all the animals.
Then do the following loop.
o Print out the round number.
o Move all the birds randomly.
o Check all the birds to see if any of them are on the same spot.
Make sure to check for touching animals AFTER moving all the animals.
o If any of the birds are touching it should print out the word “TOUCHING" to the
screen and show the names of the two animals that are touching.
• Note if A is touching B, do NOT also print that B is touching A. Each touch
should only be printed once.
o Print out the current locations and names of all birds.
Print a line "[####################################]" between each
round of the movement.
o o](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F265c74e0-bbb2-4314-bc95-c47d2c6ac651%2F610ca6c1-7227-44b3-b4aa-adfde0f29605%2Fm53eebp_processed.png&w=3840&q=75)
Transcribed Image Text:move()
When this method is run, the animal will move in a random direction.
The animal can move in one of six directions.
The animal could move left, or right, or up, or down, or in/out.
If the animal moves in one of these directions, it should move
random x number of units, where x is between 1 and 2 (inclusive).
Each direction should have an equal chance of happening, so make sure
everything is balanced.
Note that there are no parameters. The animal should self move.
Check for the range constraints.
• After the bird has moved, double check that it is still inside the box.
• If it isn't, undo the move and pick a different direction to move.
Check both x, y, and z coordinates at all times including
construction.
After the bird has moved, double check that it is still inside the box.
MAIN CLASS
Inside your main class do the following
Create an array that can hold 7 birds.
Fill the array with 4 birds with names and locations of your choice.
o Fill the array with 2 named birds but with random locations.
Finish filling the array with one default bird.
Create a touching counter that starts at zero and a round counter.
Print out the starting locations and names of all the animals.
Then do the following loop.
o Print out the round number.
o Move all the birds randomly.
o Check all the birds to see if any of them are on the same spot.
Make sure to check for touching animals AFTER moving all the animals.
o If any of the birds are touching it should print out the word “TOUCHING" to the
screen and show the names of the two animals that are touching.
• Note if A is touching B, do NOT also print that B is touching A. Each touch
should only be printed once.
o Print out the current locations and names of all birds.
Print a line "[####################################]" between each
round of the movement.
o o
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
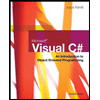
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
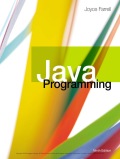
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
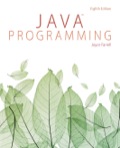
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
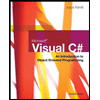
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
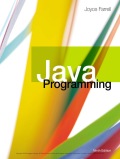
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
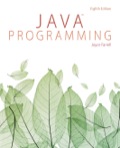
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT