mainactivity package com.example.ett; import androidx.appcompat.app.AppCompatActivity;import com.android.volley.Request; import com.android.volley.RequestQueue; import com.android.volley.Response; import androidx.appcompat.app.AppCompatActivity; import androidx.recyclerview.widget.LinearLayoutManager; import androidx.recyclerview.widget.OrientationHelper; import androidx.recyclerview.widget.RecyclerView; import com.android.volley.VolleyLog; import com.android.volley.toolbox.JsonArrayRequest;import com.android.volley.Response.Listener; import com.android.volley.toolbox.JsonObjectRequest; import java.util.List; import android.os.Bundle; import com.android.volley.AuthFailureError; import org.json.JSONArray; import org.json.JSONException; import org.json.JSONObject; import android.widget.Toast; import com.android.volley.toolbox.Volley; import org.json.JSONArray; import org.json.JSONObject; import java.util.HashMap; import java.util.Map; import java.util.ArrayList; import java.util.List; import android.os.Bundle; public class MainActivity extends AppCompatActivity { private RecyclerView recyclerView; private UniversityAdapter universityAdapter; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); recyclerView = findViewById(R.id.recycler_view); recyclerView.setLayoutManager(new LinearLayoutManager(this)); recyclerView.setHasFixedSize(true); RequestQueue requestQueue = Volley.newRequestQueue(this); String url = "http://universities.hipolabs.com/search?country=Canada"; JsonArrayRequest jsonArrayRequest = new JsonArrayRequest(Request.Method.GET, url, null, response -> { List universityList = new ArrayList<>(); for (int i = 0; i < response.length(); i++) { try { JSONObject universityObject = response.getJSONObject(i); University university = new University( universityObject.getString("name"), universityObject.getString("province"), universityObject.getString("web_pages").replace("[\"", "").replace("\"]", "") ); universityList.add(university); } catch (JSONException e) { e.printStackTrace(); } } universityAdapter = new UniversityAdapter(universityList); recyclerView.setAdapter(universityAdapter); }, error -> Toast.makeText(MainActivity.this, "Error: " + error.getMessage(), Toast.LENGTH_SHORT).show() ); requestQueue.add(jsonArrayRequest); } } ==== main .xml mainxml ----- when i run this code , the xml displays not resuit i want ,how can i fix this xml ?
mainactivity
package com.example.ett;
import androidx.appcompat.app.AppCompatActivity;import com.android.volley.Request;
import com.android.volley.RequestQueue;
import com.android.volley.Response;
import androidx.appcompat.app.AppCompatActivity;
import androidx.recyclerview.widget.LinearLayoutManager;
import androidx.recyclerview.widget.OrientationHelper;
import androidx.recyclerview.widget.RecyclerView;
import com.android.volley.VolleyLog;
import com.android.volley.toolbox.JsonArrayRequest;import com.android.volley.Response.Listener;
import com.android.volley.toolbox.JsonObjectRequest;
import java.util.List;
import android.os.Bundle;
import com.android.volley.AuthFailureError;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import android.widget.Toast;
import com.android.volley.toolbox.Volley;
import org.json.JSONArray;
import org.json.JSONObject;
import java.util.HashMap;
import java.util.Map;
import java.util.ArrayList;
import java.util.List;
import android.os.Bundle;
public class MainActivity extends AppCompatActivity {
private RecyclerView recyclerView;
private UniversityAdapter universityAdapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
recyclerView = findViewById(R.id.recycler_view);
recyclerView.setLayoutManager(new LinearLayoutManager(this));
recyclerView.setHasFixedSize(true);
RequestQueue requestQueue = Volley.newRequestQueue(this);
String url = "http://universities.hipolabs.com/search?country=Canada";
JsonArrayRequest jsonArrayRequest = new JsonArrayRequest(Request.Method.GET, url, null,
response -> {
List<University> universityList = new ArrayList<>();
for (int i = 0; i < response.length(); i++) {
try {
JSONObject universityObject = response.getJSONObject(i);
University university = new University(
universityObject.getString("name"),
universityObject.getString("province"),
universityObject.getString("web_pages").replace("[\"", "").replace("\"]", "")
);
universityList.add(university);
} catch (JSONException e) {
e.printStackTrace();
}
}
universityAdapter = new UniversityAdapter(universityList);
recyclerView.setAdapter(universityAdapter);
},
error -> Toast.makeText(MainActivity.this, "Error: " + error.getMessage(), Toast.LENGTH_SHORT).show()
);
requestQueue.add(jsonArrayRequest);
}
}
====
main .xml
mainxml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recycler_view"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="16dp"
tools:layoutManager="androidx.recyclerview.widget.LinearLayoutManager"
tools:layout_editor_absoluteX="0dp"
tools:layout_editor_absoluteY="0dp"
tools:listitem="@layout/item_university"
tools:orientation="vertical" />
<TextView
android:id="@+id/web_pages"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="aaaaaaaaaa"
tools:layout_editor_absoluteX="90dp"
tools:layout_editor_absoluteY="241dp" />
<TextView
android:id="@+id/name"
android:textSize="20dp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="TextView" />
<TextView
android:id="@+id/provance"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="488dp"
android:text="TextView"
android:textSize="30dp"
app:layout_constraintTop_toTopOf="parent"
tools:layout_editor_absoluteX="82dp" />
</androidx.constraintlayout.widget.ConstraintLayout>
-----
when i run this code , the xml displays not resuit i want ,how can i fix this xml ?

Step by step
Solved in 4 steps

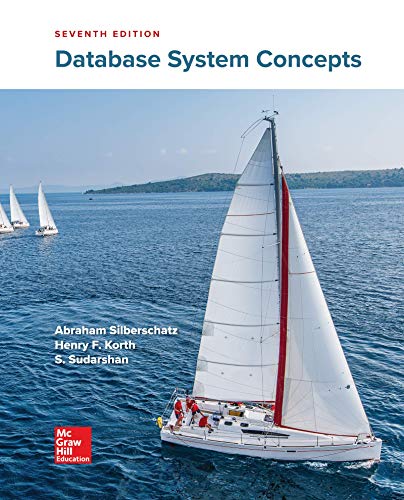
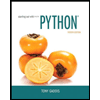
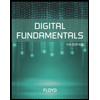
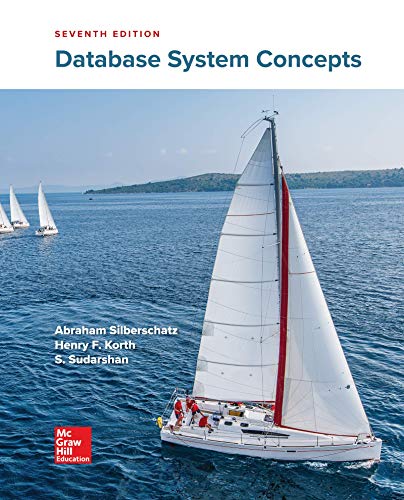
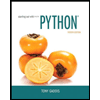
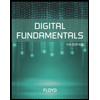
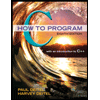
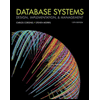
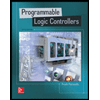