Modify the DayOfYear class, to add a constructor that takes two parameters: a string object representing a month and an integer in the range 0 through 31 representing the day of the month. The constructor should then initialize the integer member of the class to represent the day specified by the month and day of month parameters. The constructor should terminate the program with an appropriate error message if the number entered for a day is outside the range of days for the month given. Add the following overload operators: ++ prefix and postfix increment operators. These operators should modify the DayOf Year object so that it represents the next day. If the day is already the end of the year, the new value of the object will represent the first day of the year. -- prefix and postfix decrement operators. These operators should modify the DayOf Year object so that it represents the previous day. If the day is already the first day of the year, the new value of the object will represent the last day of the year.
C++ compile a program to answer question in picture included.
Code to edit:
#include <iostream>
#include <string>
class DayOfYear
{
private:
mutable int day; // Make 'day' mutable
// Static arrays to hold month names and the number of days in each month
static const std::string monthNames[];
static const int daysInMonth[];
public:
// Constructor
DayOfYear (int day) : day (day) {}
// Member function to print the day in month-day format
void
print () const
{
int tempDay = day; // Create a temporary variable
int month = 0;
// Find the month for the given day
while (tempDay > daysInMonth[month])
{
tempDay -= daysInMonth[month];
month++;
}
// Print the result
std::cout << monthNames[month] << " " << tempDay << std::endl;
}
};
// Static member variable initialization
const std::string DayOfYear::monthNames[] = {
"January", "February", "March", "April", "May", "June",
"July", "August", "September", "October", "November", "December"
};
const int DayOfYear::daysInMonth[] = {
31, 28, 31, 30, 31, 30,
31, 31, 30, 31, 30, 31
};
int
main ()
{
int userDay;
// Get user input for the day of the year
std::cout << "Enter the day of the year: ";
std::cin >> userDay;
// Validate user input
if (userDay < 1 || userDay > 365)
{
std::cerr << "Invalid input. Day of the year should be between 1 and 365."
<< std::endl;
return 1;
}
// Create an instance of the DayOfYear class with user input
DayOfYear userDayOfYear (userDay);
// Print the representation in month-day format
std::cout << "Day " << userDay << ": ";
userDayOfYear.print ();
return 0;
}


Step by step
Solved in 4 steps with 7 images

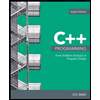
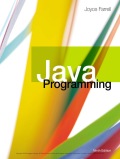
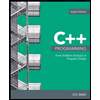
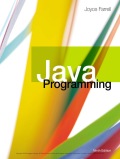