In this task, you need to use 2 priority queues to print out the kth largest and jth smallest number in 2 new different random vectors, vector3 and vector4. Write a function called int kthLargestPQ( vector& vec, int k), and a function called int jthSmallestPQ(vector& vec, int k). Here is an example: Original vector3: 9 8 9 2 3 79577 Input K for Kth largest: 3 The 3 largest number: 9 Original vector4: 9 6 5 6 7 76 162 Input J for Jth smallest: 3 The 3 smallest number: 5 Hint: Using priority queue. This problem can be solved in two ways. 1. For the kthLargestPQ, we can maintain a small heap (small priority queue, smallest element in the top) with only k elements in size. After traversing all the elements, the top element in the PQ is what we want. The jthSmallestPQ vice versa. 2. To get the kth largest element, you can construct a large priority queue using all the elements, pop the top element k-1 times, then the top element is what we want. To get the jth smallest element, you
In this task, you need to use 2 priority queues to print out the kth largest and jth smallest number in 2 new different random vectors, vector3 and vector4. Write a function called int kthLargestPQ( vector& vec, int k), and a function called int jthSmallestPQ(vector& vec, int k). Here is an example: Original vector3: 9 8 9 2 3 79577 Input K for Kth largest: 3 The 3 largest number: 9 Original vector4: 9 6 5 6 7 76 162 Input J for Jth smallest: 3 The 3 smallest number: 5 Hint: Using priority queue. This problem can be solved in two ways. 1. For the kthLargestPQ, we can maintain a small heap (small priority queue, smallest element in the top) with only k elements in size. After traversing all the elements, the top element in the PQ is what we want. The jthSmallestPQ vice versa. 2. To get the kth largest element, you can construct a large priority queue using all the elements, pop the top element k-1 times, then the top element is what we want. To get the jth smallest element, you
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 16PE:
The implementation of a queue in an array, as given in this chapter, uses the variable count to...
Related questions
Question
How does one use an priority queue to solve this type of question?

Transcribed Image Text:In this task, you need to use 2 priority queues to print out the kth largest and jth smallest number in 2
new different random vectors, vector3 and vector4. Write a function called int
kthLargestPQ(vector<int>& vec, int k), and a function called int jthSmallestPQ(vector<int>& vec,
int k).
Here is an example:
Original vector3: 9 8 9 2 3 7 9 577
Input K for Kth largest: 3
The largest number: 9
Original vector4: 9 6 5 6 7 7 6 16 2
Input J for Jth smallest: 3
The 3 smallest number: 5
Hint:
Using priority queue.
This problem can be solved in two ways.
1.
For the kthLargestPQ, we can maintain a small heap (small priority queue, smallest element in the
top) with only k elements in size. After traversing all the elements, the top element in the PQ is what we
want.
The jthSmallestPQ vice versa.
2. To get the kth largest element, you can construct a large priority queue using all the elements, pop
the top element k-1 times, then the top element is what we want. To get the jth smallest element, you

Transcribed Image Text:2. To get the kth largest element, you can construct a large priority queue using all the elements, pop
the top element k-1 times, then the top element is what we want. To get the jth smallest element, you
can construct a small priority queue using all the elements, pop the top element j-1 times, then the top
element is what we want.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
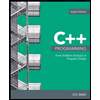
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
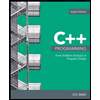
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning